수정(추가)
www.notion.so/neneee/mybatis-a64891971a4b4b6e82cf79a8c8061273
mybatis 사용 방법
mybatis 사용 방법
www.notion.so
이러한 방법이 훨신 더 편하고 깔끔한거 같다.(두개다 참고)
dependencies {
implementation 'org.springframework.boot:spring-boot-starter-web'
implementation 'org.mybatis.spring.boot:mybatis-spring-boot-starter:2.1.3'
runtimeOnly 'mysql:mysql-connector-java'
testImplementation('org.springframework.boot:spring-boot-starter-test') {
exclude group: 'org.junit.vintage', module: 'junit-vintage-engine'
}
}
Spring boot 에서 mybatis를 빌드 받는다.
application.properties에 mysql을 연동할 정보를 입력한다.
spring.datasource.driver-class-name=com.mysql.cj.jdbc.Driver
spring.datasource.url=jdbc:mysql://localhost:3306/[database이름]?serverTimezone=UTC&useUnicode=true&charaterEncoding=utf-8
spring.datasource.username=[유저 이름]
spring.datasource.password=[유저 비밀번호]
필자는 여기서 유저의 권한을 줘야하는데 안줘서 시간을 좀 날렸다. db에 접속할 수 있도록 새로 생성한 유저에게 권한을 꼭 주자.
com.example.demo.dao.UserDTO
package com.example.demo.dto;
public class UserDTO {
private int seq;
private String name;
private String country;
public UserDTO(int seq, String name, String country) {
this.seq = seq;
this.name = name;
this.country = country;
}
public int getSeq() {
return seq;
}
public void setSeq(int seq) {
this.seq = seq;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getCountry() {
return country;
}
public void setCountry(String country) {
this.country = country;
}
}
lombok를 사용해도 좋다.
com.example.demo.dao.UserDAO
package com.example.demo.dao;
import com.example.demo.dto.UserDTO;
import org.springframework.stereotype.Component;
import java.util.List;
@Component
public interface UserDAO {
List<UserDTO> selectUsers(UserDTO param) throws Exception;
}
DAO를 interface로 선언해준다.
package com.example.demo;
import org.apache.ibatis.session.SqlSessionFactory;
import org.mybatis.spring.SqlSessionFactoryBean;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.context.annotation.Bean;
import org.springframework.core.io.Resource;
import org.springframework.core.io.support.PathMatchingResourcePatternResolver;
import javax.sql.DataSource;
@SpringBootApplication
public class DemoApplication {
public static void main(String[] args) {
SpringApplication.run(DemoApplication.class, args);
}
@Bean
public SqlSessionFactory sqlSessionFactory(DataSource dataSource) throws Exception {
SqlSessionFactoryBean sessionFactory = new SqlSessionFactoryBean();
sessionFactory.setDataSource(dataSource);
Resource[] res = new PathMatchingResourcePatternResolver().getResources("classpath:mappers/*Mapper.xml");
sessionFactory.setMapperLocations(res);
return sessionFactory.getObject();
}
}
Application 클래스에서 초기화를 해준다.
com.example.demo.controller.UserController
package com.example.demo.controller;
import com.example.demo.dao.UserDAO;
import com.example.demo.dto.UserDTO;
import org.mybatis.spring.annotation.MapperScan;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestParam;
import org.springframework.web.bind.annotation.RestController;
import java.util.List;
@RestController
@MapperScan(basePackages = "com.example.demo.dao")
public class UserController {
@Autowired
private UserDAO userDAO;
@RequestMapping("/users")
public List<UserDTO> users(@RequestParam(value = "country", defaultValue = "") String country) throws Exception {
final UserDTO param = new UserDTO(0, null, country);
final List<UserDTO> userList = userDAO.selectUsers(param);
return userList;
}
}
컨트롤러에 입력을 해준다.
resources.mappers.UserMapper.xml
<?xml version="1.0" encoding="UTF-8"?>
<!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN"
"http://mybatis.org/dtd/mybatis-3-mapper.dtd">
<mapper namespace="com.example.demo.dao.UserDAO"><!--namespace를 통해 UserDAO와 연결합니다. -->
<select id="selectUsers" parameterType="com.example.demo.dto.UserDTO"
resultType="com.example.demo.dto.UserDTO"><!-- selectUsers()메서드를 호출하면 실행할 SELECT문이며,
UserDTO 객체를 파라미터로 받아, UserDTO객체를 반환합니다.-->
SELECT `seq`, `name`, `country`
FROM lab03
<if test='country != null and country != ""'><!-- 만약 파라미터에 country가 있다면 SQL문에 WHERE절을 추가합니다. -->
WHERE country = #{country}
</if>
</select>
</mapper>
결과를 확인해주기 위해 Postman을 사용한다.
System.println.out을 사용해도된다.
Postman | The Collaboration Platform for API Development
Postman makes API development easy. Our platform offers the tools to simplify each step of the API building process and streamlines collaboration so you can create better APIs faster.
www.postman.com
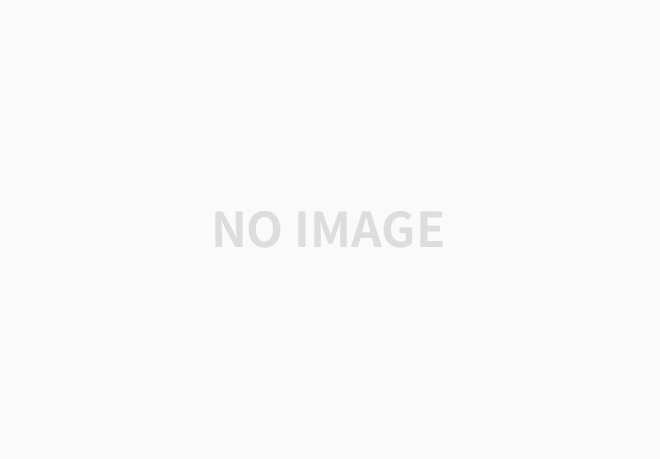
결과 화면이다.
웹사이트에서 실행하면 아마 cross domain error에 걸리니 어플리케이션을 깔아서 해보도록하자.
여기서 보고 따라 정리했다.
Spring-boot : MyBatis를 이용하여 MySQL 연동하기
MyBatis를 사용하는 이유? 우선 MyBatis를 알기 전에 JDBC를 먼저 알아야한다. 간단하게 JDBC는 Java에서 DB연동하기 위한 API다. 이 JDBC만 사용하게 되면 Java소스 와 Query소스가 겹치게 되고 관리가 어려워
velog.io
따라하다보면 안되는 부분은 필자에 적혀있는거 보고 따라치면 된다.
'코딩 > Spring' 카테고리의 다른 글
JPA 저장을 했는데, 데이터가 없는 경우 (0) | 2022.11.10 |
---|---|
Spring ajax json 및 데이터 가져오기 (1) | 2022.02.14 |
UnsatisfiedDependencyException,BeanCreationException,IllegalArgumentException [spring boot 에러] (1) | 2021.08.15 |
Spring message properties 메시지 설정하는 법 (1) | 2020.09.16 |